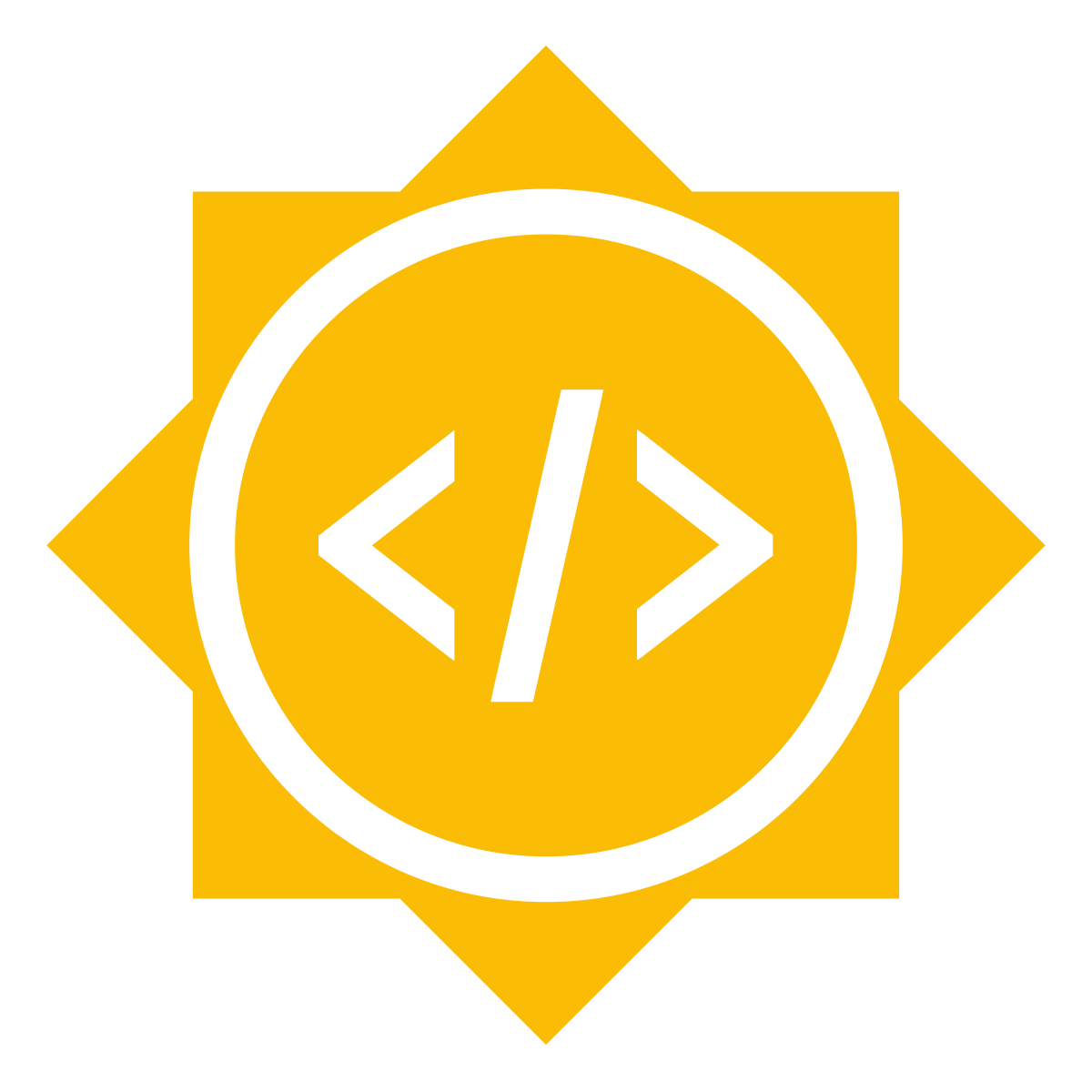

p5-teach.js
Project Abstract
This project would involve developing tools for teaching STEM through p5.js, adding functions to animate shapes, and animating maths symbols. The main focus is to introduce a simple, easy-to-use library to animate repetitive methods. It would provide educators tools to make interactive animated sketches that can support learning in the remote environment. It will take advantage of the p5.js core library - p5.sound.js for sound effects and KaTeX for rendering symbols.
Project Description
Overview
- Scenes and transitions
- Transform functions
- TeX editor
- Create a player that can tell progress from 0% to 100% over time and control the scene
- play(), pause() and stop()
- Typing effects
- fadeIn, fadeOut, blink, wipeIn, wipeOut, zoomIn, zoomOut
- Sound/Audio support
- Camera controls (like manim.scene.moving_camera_scene)
- Feedback from educators
- Unit tests (using mocha and chai)
Detailed
Why p5-teach.js?
Problems faced by the teachers of high school and professors of STEM:
- The main problem for STEM teachers is installation and setup.
- Teachers of high schools use tools such as Desmos and GeoGebra for visualization but can’t be used for explaining derivations involving a lot of math symbols.
- Professors use MATLAB for visualizations and it is not easy to learn a new language considering their time constraints.
- They don’t have access to proper documentation for animation engines for mathematics.
- They need transition and scenes but during installation and setup of animation engine (like manim), they get demotivated and get back to MATLAB
- The visualizations they are using are not pretty.
- STEM teachers need LaTeX for work but it is not flexible. For example, they want to label graphs with maths symbols but it is not easy to do so.
- All STEM teachers are not necessarily good coders. They don’t want to use software that is very tough to learn, as it will take some time and a learning curve to get familiar with.
- It is not easy to tweak the library because of the complex coding concepts used in the library.
p5-teach.js will try to resolve these problems.
Key-features:
- Easy to set up and ready-to-use examples
- Getting started guides and cheat sheets for beginners
- It would not be tough for beginners to learn
- It will be pretty and will support transitions
- Documentation and examples
p5-teach.js will broaden the community by connecting with more people from the STEM field. Bringing people from STEM fields will be a step to follow our commitments towards adding features to p5.js that increase access. We focus on making p5.js available to and approachable for people who are excluded from the p5.js community (intentionally or not) and from similar tools and communities. The community will benefit from the involvement of more STEM teachers and can explore together more art, science, and technology.
The project can be divided into three stages:
- Development of animation methods, scene control, and tools
- Adding support for KaTeX and sound in animations
- Testing and Documentation
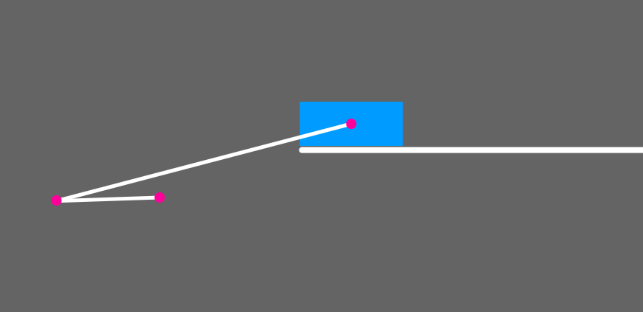
This is an example of animation ported from the manim (python) library into a p5.js sketch. In manim and other animation engines, it is easy to export high-quality video but they are very tedious to set up. Maths require symbols that we usually make from LaTeX tools which are not easy to integrate into animations. The power of p5.js lies in its beginner-friendly nature which will help to solve these issues. We can build a library to solve these problems.
The primary aim is to provide listed tools for teachers who just started with p5.js and javascript
- Animation through browser
- TeX editor
Animation through browser
This feature includes animation playing within the browser but also allowing users to interact while the animation is playing, such as a player that can tell progress from 0% to 100% over time and control the scene. We can add sound and speed controls to make the user experience more interactive. Educators can embed their sketches in webpages or can share links to their students to make the distance learning experience more interactive.
Such as

This is an example of animation through the web browser. https://editor.p5js.org/radium.scientist/present/aOz6FS2j_
Typing Effects

Typing effects can be manually produced but are difficult to do for large sentences and will be monotonous. We can inspire from manim and can develop text animations such as the following:

TeX editor
Why TeX editor?
TeX editor is required for rendering KaTeX to the canvas in creative ways so that educators (specifically those who post mathematical problems through the online medium in form of Google quiz, Instagram post, or Twitter) can screenshot (with inbuilt tools such as snipping tool in Windows 10 or open-source tools such as ShareX) and share them online. TeX editor can change the background, font color, load fonts, resize, drag and drop TeX. We can try to build features to export as SVG or PNG by using canvas-latex library that renders KaTeX to canvas and then we can use toDataUrl() methods to create an image to export SVG or PNG from TeX editor, but it can take a lot of time which we can invest on other features this summer.
https://two-ticks.github.io/p5-teach.js/katex-editor/

TeX editor is similar to Maths in Motion.
This project is more focused on educators using online mediums to teach. Many educators post on Twitter, Instagram, and YouTube, TeX editor will help them build posts by providing them tools to work with KaTeX easily.
ShareX or OBS Studio can be used to record animations and capture screenshots for posting online (in the future we can add exporting features with help of canvas-latex). We can add examples for recording with the help of CCapture library (this will not be able to record KaTeX elements because KaTeX is added as a DOM element but such libraries record only canvas).
API
Function |
Description |
write() |
Writes the text with a blurry effect at each new character. |
typeWriter(object) |
Write the text with the typing effect. |
fadeIn(object, duration) |
Make a fade in effect. |
fadeOut(object, duration) |
Make a fade out effect. |
blink(object, duration) |
Make a blinking effect. |
wipeIn(object, duration) |
Make a wipe in effect. |
wipeOut(object, duration) |
Make a wipe out effect. |
zoomIn(object, duration) |
Make a zoom in effect. |
zoomOut(object, duration) |
Make a zoom out effect. |
moveAlongPath(object, path) |
Moves object along the defined path |
Transform(object1, object2) |
Transforms one shape into another by interpolating vertices from one to another. Similar to this example |
Shift(x,y) |
Shifts shape and object by x and y |
wait(duration) |
Waits for the duration specified before playing the next animation. It is helpful in timing the animation. |
Create(object) |
Creates animation of object building up from scratch. |
Additional Features (to be discussed)
- 3-D shapes and interactions using three.js or p5-WebGL
- Export as SVG or PNG from TeX editor
Unit Tests
We will use chai for making our test cases and mocha for driving our test cases.
Work done so far