Note that you can also build these out of map() if you want them to be sort of like max/msp or pd components.
So, say instead of a triangle wave you wanted an s-curve (sigmoid function):
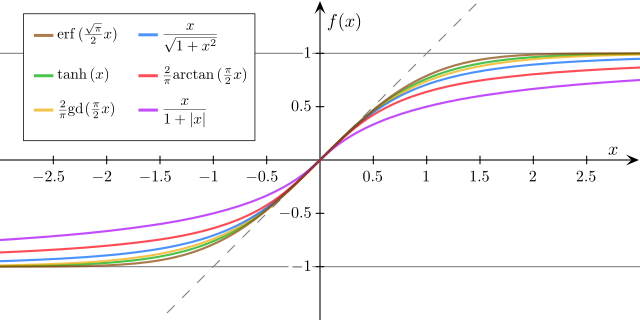
So you want your component to do this:
1/(1 + e^-x)
which is written:
1/(1 + exp(-x))
But you need to map ranges of inputs and outputs around it…
Below are some simple examples of wrapping these kinds of things up in a convenient function using map().
/**
* map-based waves
* Processing 3.4 2019-08
* https://discourse.processing.org/t/variable-to-read-an-array-of-numbers-in-p5/13377
*/
void draw() {
background(128);
float y=0;
for(float x=0; x<width; x=x+0.2){ // loop over screen to draw points
y = mapSawtooth(x, 0, 10, 100, 70); // period of 1-10, output 100-70
point(x, y);
y = mapTriangleWave(x, 0, 50, 35, 65);
point(x, y);
y = mapSigmoid(x, 0, width, 30, 5);
point(x, y);
}
}
/**
* call a generic wave / saw / square function.
* Use map to put x in; use map to get y out.
**/
float mapSigmoid(float x, float start1, float stop1, float start2, float stop2){
float nx=map(x, start1, stop1, -TWO_PI, TWO_PI);
float y = sigmoid(nx);
float ny = map(y, 0, 1, start2, stop2);
return ny;
}
/**
* s-curve: sigmoid generic formula.
* Over x[-TWO_PI, TWO_PI] its output y ranges [0-1]
**/
float sigmoid(float x) {
return 1/(1 + exp(-x));
}
float mapTriangleWave(float x, float start1, float stop1, float start2, float stop2){
float nx=map(x, start1, stop1, 0, 1);
float y = triangleWave(nx);
float ny = map(y, 0, 1, start2, stop2);
return ny;
}
/**
* triangle wave generic formula.
* Over x[0, 1] its output y ranges [0-1-0]
**/
float triangleWave(float x) {
return 2 * abs(sawtooth(x)-0.5);
}
float mapSawtooth(float x, float start1, float stop1, float start2, float stop2){
float nx=map(x, start1, stop1, 0, 1);
float y = sawtooth(nx);
float ny = map(y, 0, 1, start2, stop2);
return ny;
}
/**
* sawtooth wave
* Over x[0, 1] its output y ranges [0-1]
*/
float sawtooth(float x){
return x % 1.0;
}

The key idea is that you are taking a really simple piece of code – like x % 1.0
for a sawtooth – and wrapping it in a pretty generic way to turn it into something like a pd component.